- Java Program Grocery Receipt Example
- Java Program Grocery Receipt Template
- Java Program Grocery Receipts
- Java Program Grocery Receipt Examples
System presents receipt. Customer leaves with receipt and goods (if any). Suppose you are asked to write a program to automate the above steps. Your assignment 1 is to write such a program in Java. You are not required to use sophisticated GUI for user interaction but to use simple console text interaction. Find more on Program to display bill receipt based on customer information and create telephone directory Or get search suggestion and latest updates. Easy Tutor author of Program to display bill receipt based on customer information and create telephone directory is from United States. I need a java program that consists of 2 files: one with the methods and the other one to display using JOptionpane a grocery receipt only. The file with the methods must contain: Quantity, Item#, and read more.
There are several methods to create/generate invoice /bill/receipt in java. In this tutorial show you, how to create/generate invoice /bill/receipt in java using Textarea. Using this method you can generate an invoice for any kind of java project. This tutorial will help to develop a point of sales system. Here show you how to add sales details to the invoice and how to print the invoice. You can follow the video tutorial and source code.
Features and functions of this simple application
Bill/Invoice header section

In here create invoice header using simple java code. You can add names, contact numbers, address and other details to bill/invoice header when you develop invoice. This section develops as a static section.
Bill/ Invoice Details section
In this section include dynamic details regarding the invoice. Example, when we create sale invoice, can add sales item details, price, cash, total and more details to this section.
Footer section
This section used as a static section. We can add anything to modify the invoice.

We can print this invoice using the print method in java.
Source code of create/generate invoice /bill/receipt in java project
The technologies used in create/generate invoice /bill/receipt in java project
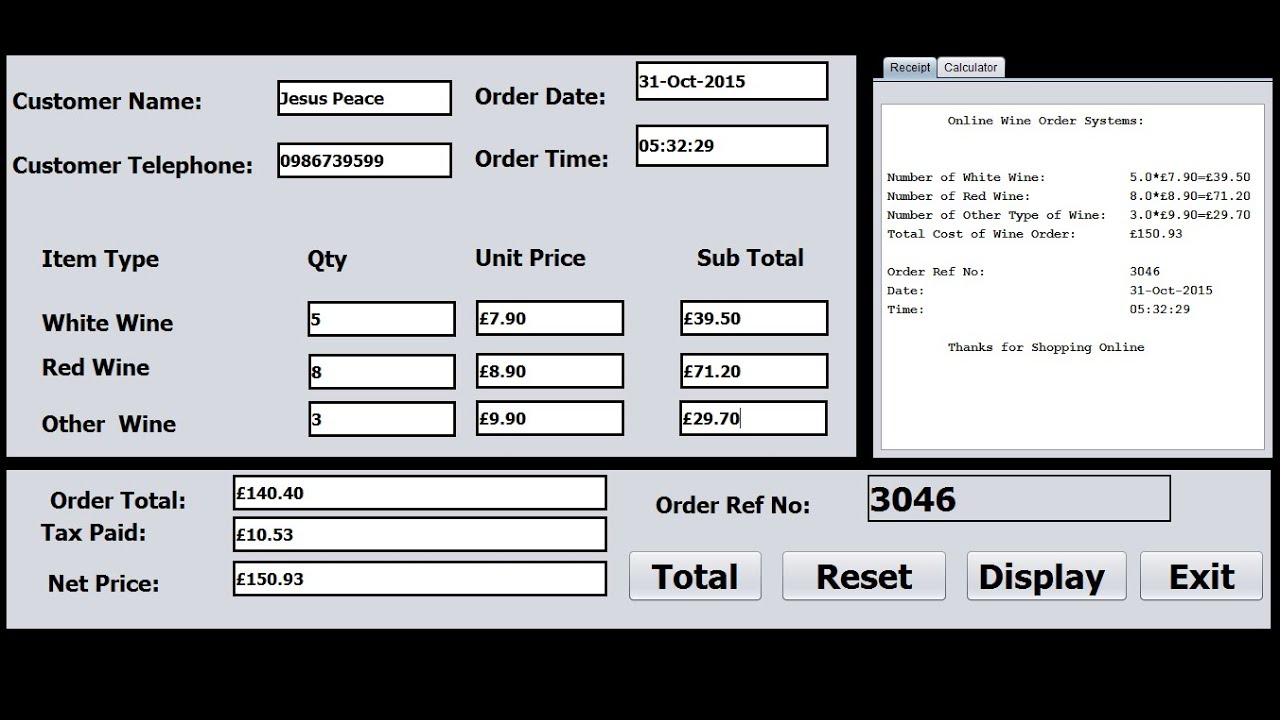

Java Program Grocery Receipt Example
- Java: All codes have been written using the java programming language.
- Netbeans: This project has developed inside of the Netbeans IDE.
How to use this
You can download the complete project file and database using the below link.
Java Program Grocery Receipt Template
The goals of providing this project:
- To provide an example for students to develop their own projects.
- helping people create their projects.
- sharing knowledge and codes for educational purpose.
This project is not for:
- You cannot use this project or project source codes for commercial purposes
- You cannot re-upload this project or project source code to web internet or any kind of space.
Do you need help to remotely set up my any project on your machine or customize any project with your requirement please contact syntech1994@gmail.com
Java Program Grocery Receipts
Copyright © codeguid
Receipt Calculator Simulation
Java Program Grocery Receipt Examples
Good.javapackage receipts;import java.text.DecimalFormat;// Class representing good in the shop basket. Stores number of this good in the basket, price of one instance of this good.public class Good {// Keywords for determination if good is exempt or notprivate static final String[] exemptKeyWords = {'food', 'book', 'medicine', 'chocolate', 'pill'};// Format for outputting decimal numbersprivate static DecimalFormat df = new DecimalFormat('0.00');// Instance private fields of classprivate String name;private double price;private int number;private boolean isImported;private boolean isExempt;public Good(String name, double price, int number) throws IllegalArgumentException{// Throwing exceptions if parameters are illegalif (price <= 0.0) {throw new IllegalArgumentException('Price must be positive');}if (number <= 0.0) {throw new IllegalArgumentException('Quantity must be positive integer');}this.name = name;this.price = price;this.number = number;isImported = false;isExempt = false;// Checking if good is imported or exemptString lcName = name.toLowerCase();if (lcName.contains('imported')) {isImported = true;}// If name of good contains one of keywords, then good is exemptfor (String exemptKeyWord : exemptKeyWords) {if (lcName.contains(exemptKeyWord)) {isExempt = true;break;}}}public Good(String name, double price) {this(name, price, 1);}// Getter for name fieldpublic String getName() {return name;}// Adding more instances of the same good (increasing number field of the class).// If prices differ, returns false.public boolean addQuantity(Good good) {if (this.price != good.price) {return false;}this.number += good.number;return true;}// Checking if name of the current good matches given namepublic boolean equals(String name) {return this.name.equals(name);}// Return overall sale tax for 'number' of goodspublic double getTax() {if (isExempt) {return 0.0;}return number*Math.ceil((price*0.1)/0.05)*0.05;}// Return overall import tax for 'number' of goodspublic double getImportTax() {if (!isImported)return 0.0;return number*Math.ceil((price*0.05)/0.05)*0.05;}// Calculates overall price for 'number' of goods (including taxes)public double getOverallPrice() {return number*price + getTax() + getImportTax();}// Returns the string representation of good info.public String toString() {StringBuffer buf = new StringBuffer();buf.append(name + ': ' + df.format(getOverallPrice()));if (number >1) {buf.append(' (' + number + ' @ ' + df.format(getOverallPrice()/number) + ')');}return buf.toString();}}Receipt.javapackage receipts;import java.text.DecimalFormat;import java.util.ArrayList;import java.util.InputMismatchException;import java.util.List;import java.util.NoSuchElementException;import java.util.Scanner;// Class representing selected set of goods.public class Receipt {// Format for outputting decimal numbersprivate static DecimalFormat df = new DecimalFormat('0.00');// List, containing all selected goodsprivate List basket;private double totalPrice;private double taxes;public Receipt() {basket = new ArrayList<>();taxes = 0;}// Adding good to the basket. If no such kind of good was selected previously, than adding new good instance to the basket list.// Otherwise, increasing number field in corresponding good instance.public void addGood(Good good) throws IllegalArgumentException{for (Good g : basket) {if (g.equals(good.getName())) {if (!g.addQuantity(good)) {throw new IllegalArgumentException('Two goods with the same name but different data are found.');}return;}}basket.add(good);}// This method outputs all necessary infopublic void outputAll() {for (Good g : basket) {// Cumulating total overall cost and taxestaxes += (g.getTax() + g.getImportTax());totalPrice += g.getOverallPrice();// Printing separate good infoSystem.out.println(g);}System.out.println('Sales taxes: ' + df.format(taxes));System.out.println('Total price: ' + df.format(totalPrice));}// Main method. Reads items from console, until empty string is enteredpublic static void main(String[] args) {Scanner scanner = new Scanner(System.in);Receipt receipt = new Receipt();// Reading from consolewhile(true) {// If line is empty, then quitString line = scanner.nextLine();if (line.equals(')) {break;}// Scanning entered lineScanner lineScan = null;try {// Line must contain word 'at'int index = line.lastIndexOf('at');if (index -1) {throw new IllegalArgumentException('Illegal input format: no 'at' found.');}// Reading price after word 'at'. Can throw NumberFormatExceptionString priceLine = line.substring(index+2);double price = Double.parseDouble(priceLine);lineScan = new Scanner(line.substring(0, index));// Reading item quantity in the beginning of the line. Can throw InputMismatchExceptionint number = lineScan.nextInt();// Trying to read good name. NoSuchElementException can be thrown if name is empty.String name = lineScan.next();while (lineScan.hasNext()) {name += (' ' + lineScan.next());}// Creating new item instance and putting it to the basketreceipt.addGood(new Good(format(name), price, number));lineScan.close();}// Processing errorscatch(NumberFormatException nfe) {System.out.println('Can not read price');}catch (InputMismatchException ime) {System.out.println('Can not read quantity');lineScan.close();}catch (NoSuchElementException nsee) {System.out.println('Good item name is empty');lineScan.close();}catch(IllegalArgumentException iae) {if (lineScan != null) {lineScan.close();}System.out.println(iae.getMessage());}}scanner.close();// Printing resultsreceipt.outputAll();}// Formatting good name. First letter of it must be capital.private static String format(String word) {return word.substring(0,1).toUpperCase() + word.substring(1);}}